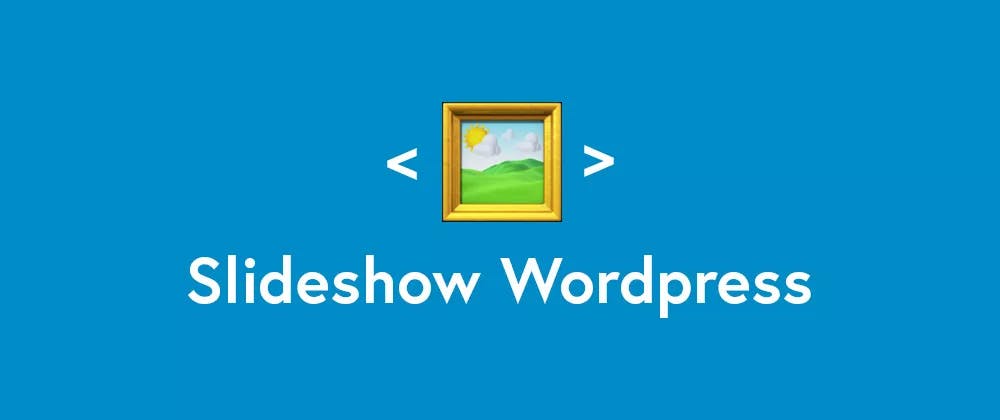
How to build a simple Wordpress slidehow in php with JavaScript and CSS 🖼
Hi guys, today I want to show you how to create a simple slideshow in wordpress without using plugins for it.
In my example I use a wordpress query to get all the images of the last five posts.
This is what the slider looks like when it's done:
PHP and HTML part
If you're wondering where to put this code, here's how I did it. I wanted to have the slideshow on the front page of the wordpress site. Therefore I have to put the code in the front-page.php
of my template.
First of all we want to get the 3 last posts, Wordpress fortunately already provides a Query function for that:
<?php $last_three_posts = new WP_Query('posts_per_page=3');?>
<?php $last_three_posts = new WP_Query('posts_per_page=3');?>
The parameter post_per_page=3
indicates that we only want the last three posts. We then store these in the variable $last_three_posts
.
Now we can loop through all the posts and display the thumbnail image of each post.To do this we can use a foreach loop:
foreach ($the_query->get_posts() as $post): ?> <img src=<?php echo get_the_post_thumbnail_url($post)?> alt=<?php echo $post->post_title ?> style="width:100%"> <?php endforeach; ?>
foreach ($the_query->get_posts() as $post): ?> <img src=<?php echo get_the_post_thumbnail_url($post)?> alt=<?php echo $post->post_title ?> style="width:100%"> <?php endforeach; ?>
The function get_the_post_thumbnail_url($post)
is also provided by wordpress. With this function we get the url to the image of the respective post. If you run this code, the result should look something like this:
Styling
Style the next and previous buttons, the caption text and the dots:
<style> .mySlides { display: none; } .fade { animation-name: fade; animation-duration: 1.5s; } #slideshow { position: relative; } #previmg { position: absolute; top: 50%; left: 0; } #nextimg { position: absolute; top: 50%; right: 0; } #text { position: absolute; bottom: 16px; text-align: center; width: 100%; color: white; background-color: #ffccee2e; backdrop-filter: blur(10px); padding: 8px; } #numbertext { position: absolute; top: 16px; left: 16px; padding: 8px; color: white; background-color: #ffccee2e; backdrop-filter: blur(10px); border-radius: 50%; } @keyframes fade { from {opacity: .4} to {opacity: 1} } </style>
<style> .mySlides { display: none; } .fade { animation-name: fade; animation-duration: 1.5s; } #slideshow { position: relative; } #previmg { position: absolute; top: 50%; left: 0; } #nextimg { position: absolute; top: 50%; right: 0; } #text { position: absolute; bottom: 16px; text-align: center; width: 100%; color: white; background-color: #ffccee2e; backdrop-filter: blur(10px); padding: 8px; } #numbertext { position: absolute; top: 16px; left: 16px; padding: 8px; color: white; background-color: #ffccee2e; backdrop-filter: blur(10px); border-radius: 50%; } @keyframes fade { from {opacity: .4} to {opacity: 1} } </style>
Javascript
<script> let i; let slideIndex = 0; let slides = document.getElementsByClassName("mySlides") slides[slideIndex].style.display = "block" let nextimg = document.getElementById('nextimg') let previmg = document.getElementById('previmg') let numbertext = document.getElementById('numbertext') numbertext.innerText = `1 / ${slides.length}` showSlides() nextimg.addEventListener('click', () => { if (slides.length-1 != slideIndex) { slideIndex++ } numbertext.innerText = `${slideIndex + 1} / ${slides.length}` for (let j = 0; j < slides.length; j++) { if (j == slideIndex) { slides[slideIndex].style.display = "block" } else { slides[j].style.display = "none" } } }) previmg.addEventListener('click', () => { if (slideIndex != 0) { slideIndex-- } numbertext.innerText = `${slideIndex + 1} / ${slides.length}` for (let j = 0; j < slides.length; j++) { if (j == slideIndex) { slides[slideIndex].style.display = "block" } else { slides[j].style.display = "none" } } }) function showSlides() { let j; let slides = document.getElementsByClassName("mySlides"); for (j = 0; j < slides.length; j++) { slides[j].style.display = "none"; } slideIndex++; if (slideIndex > slides.length) {slideIndex = 1} slides[slideIndex-1].style.display = "block"; setTimeout(showSlides, 5000); // Change image every 2 seconds } </script>
<script> let i; let slideIndex = 0; let slides = document.getElementsByClassName("mySlides") slides[slideIndex].style.display = "block" let nextimg = document.getElementById('nextimg') let previmg = document.getElementById('previmg') let numbertext = document.getElementById('numbertext') numbertext.innerText = `1 / ${slides.length}` showSlides() nextimg.addEventListener('click', () => { if (slides.length-1 != slideIndex) { slideIndex++ } numbertext.innerText = `${slideIndex + 1} / ${slides.length}` for (let j = 0; j < slides.length; j++) { if (j == slideIndex) { slides[slideIndex].style.display = "block" } else { slides[j].style.display = "none" } } }) previmg.addEventListener('click', () => { if (slideIndex != 0) { slideIndex-- } numbertext.innerText = `${slideIndex + 1} / ${slides.length}` for (let j = 0; j < slides.length; j++) { if (j == slideIndex) { slides[slideIndex].style.display = "block" } else { slides[j].style.display = "none" } } }) function showSlides() { let j; let slides = document.getElementsByClassName("mySlides"); for (j = 0; j < slides.length; j++) { slides[j].style.display = "none"; } slideIndex++; if (slideIndex > slides.length) {slideIndex = 1} slides[slideIndex-1].style.display = "block"; setTimeout(showSlides, 5000); // Change image every 2 seconds } </script>