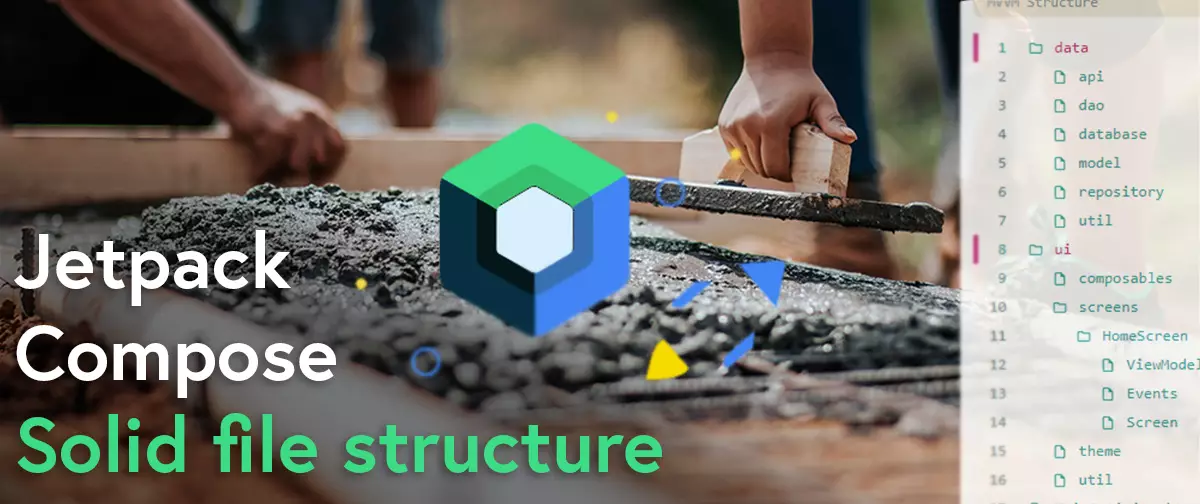
🏗 Kotlin String Formatting with Examples
In Kotlin, String is a common data type used in almost every code project. This means that it’s important for programmers to know how to format them correctly, such as how to run code within a string.
Even if string formatting is not difficult, there are painfully many methods to do it.
This is a complete guide for string formatting in Python.
3 Ways to Format Strings in Kotlin
val someValue = 1
val v1 = "The value is: $someValue"
val v2 = "The value is: ${someValue}"
val v3 = String.format("The value is: %s", someValue)
This table summarizes the string formatting options in Kotlin.
Method | Example |
---|---|
$ Operator | "The value is: $x" |
${x} Operator | "The value is: ${x}" |
String.format() | "The value is: %s".format("x") |
1. String templates with the "$" dollar symbol
The modern versions of Kotlin introduced another method for formatting strings that is even more intuitive and readable than the String.format()
method. The new method for formatting strings is called String-Literals. A template expression starts with a dollar sign ($) and consists of either a name or an expression in curly braces
String literals let you bypass the inconvenient .format()
method and insert values and expressions directly into strings by enclosing them in curly braces. To create a string literal, all you have to do is put a dollar sign in front of the expression.
Here’s an example that gives you an idea of an f-string in Python:
fun main() {
val name = "Bob"
val sentence = "Hi, $name"
println(sentence)
}
Output in the console:
Hi Bob
Syntax
Here’s the generic syntax for using the String-Literals in Kotlin:
"$val"
Here the dollar sign tells the Kotlin interpreter that it will be a formatted string literal. val is a value or expression that Kotlin executes and inserts into the string.
Example 2. Inserting multiple values into a string
fun main() {
val her_name = "Alice"
val my_name = "Bob"
val my_age = 21
val sentence = "Hi, $her_name. I'm $my_name and I'm $my_age years old."
println(sentence)
}
Output in the console:
Hi, Alice. I'm Bob and I'm 21 years old!
2. String templates with the ${x}
dollar symbol
The curly brackets extend the function of the string literals even more. Inside of thes brackets Kotlin code can be executed. Here an example:
fun main() {
val n = 1
val msg = "n + 1 = ${n + 1}"
println(msg)
}
Output in the console:
n + 1 = 2
Unlike Java, many of the constructs in Kotlin are expressions. For this reason, a string template can also contain logic:
fun main() {
val n = 1
val msg = "$n is ${if(n > 0) "positive" else "not positive"
println(msg)
}
Output in the console:
1 is not positive
3. String templates with .format() funciton
fun main() {
val textOne = "This"
val textTwo = "Welcome"
var textThree = textOne
textThree += " is"
textThree += " a long text"
println("Format:\n%s\n%s"
.format(textOne, textThree))
}
The output in the console looks like this:
Formatieren:
This
This is a long text
The three variables textOne
, textTwo
and textThree
are assigned values. The variable textThree
initially receives the value of the variable textOne
.
The +=
operator is used to extend the value of a string variable. This means that further strings are appended.
The value of a string (or a string variable) can be shaped using the format()
method. In this way, variables of different types can be embedded in a string.
Placeholders are used. Placeholders of type %s are used to embed string variables or strings.
The round brackets after the name of the format() method contain parameters. They are embedded in the string one after the other in the place of each placeholder. Make sure that both the number and the order of the parameters and the placeholders match.
In this way, a longer string is assembled. The assignment of this string results in the output on the console. Here's a full list of specifier types for the format function you can use in Kotlin:
Datatype | Specifier |
---|---|
%b | Boolean |
%c | Character |
%d | Signed Integer |
%e | Float in Scientific Notation |
%f | Float in Decimal Format |
%g | Float in Decimal or Scientific Notation |
%h | Hashcode of the supplied argument |
%n | Newline separator |