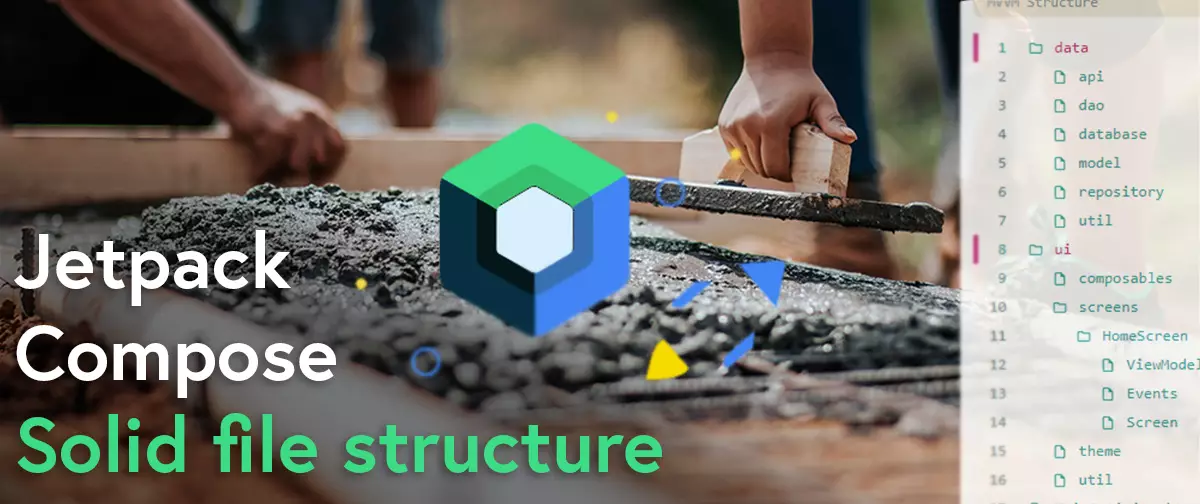
🏗 How to structure your jetpack compose project
Jetpack Compose is a powerful library for creating user interfaces in Android apps. It provides a modern, declarative way of designing user interfaces and simplifies the process of creating complex layouts.
I had difficulty setting up a suitable folder structure for Jetpack Compose initially. To help others avoid this problem, I've summarized some folder structures for different project approaches in this blog post.
We'll explore the file structure of Jetpack Compose and the best practices for organizing code. These approaches may not always fit perfectly, but they can provide a good starting point and a general guideline.
File structure for MVVM
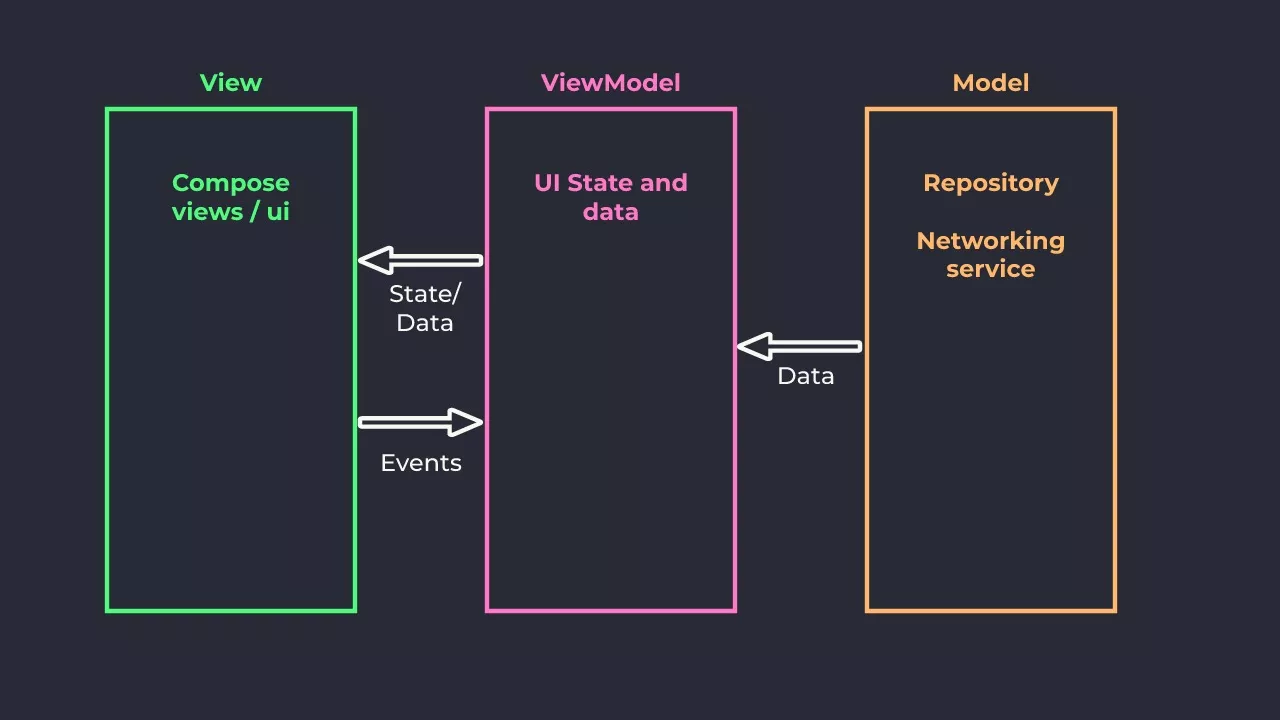
The first folder structure is the one I use the most, it uses the Model View ViewModel design pattern.
Model View ViewModel (MVVM) is an architectural pattern for creating user interfaces in Android apps. It is based on the Model-View-Controller architecture, but it is optimized for Android development. MVVM allows developers to separate the logic and data of their app from the UI, which makes it easier to test and debug code.
In this example we splited the app in two parts once in Ui (line 8) and once in Data (line 1). I think this folder structure works for small projects as well as for bigger ones, because it scales well.
The Data
folder holds the models (line 5), the Ui
folder contains the views (line 14) and the ViewModel (line 12).
All of these file structures are only examples; they can be customized.
Alternative for smaller projects
This is an excellent example of a file structure for a smaller project with a manageable level of complexity, such as a single activity application. I sourced this example from the JetChat Github repo, which is just one of the many examples available. It is highly recommended to take a peek at the repo and the code; many of the features can easily be adapted to your own project. Furthermore, the JetChat repo offers a great insight into how to structure a project and how to organise the code. Additionally, you can learn from the example how to make the code more efficient and how to use best practices when it comes to coding.
Clean Architecture project structure
Clean Architecture is a modern software design approach that enables developers to create maintainable and scalable codebases. It emphasizes the separation of concerns and encourages developers to build modular and decoupled systems.
By using a clean architecture, you can develop applications with very little coupling and independent of technical implementation details such as databases and frameworks. This way, the application becomes easy to maintain and flexible to change. It also becomes inherently testable.
The Core Principles of Clean Architecture
Clean Architecture is based on the core principles of separation of concerns, dependency inversion, and the single responsibility principle. These principles enable developers to create maintainable codebases that are easier to understand and less prone to errors.
Here is an example of how a clean architecture Jetpack Compose project's file structure might look like:
Here is a small explanation of what each layer means:
Data (line 4)
: All data sources are defined in the broadest sense.Area (line 7)
: The business logic of the application is stored here.Use cases (line 10)
: Interactors are another name for them.Presentation (line 12)
: This is a layer that interacts with the (UI) user interface.