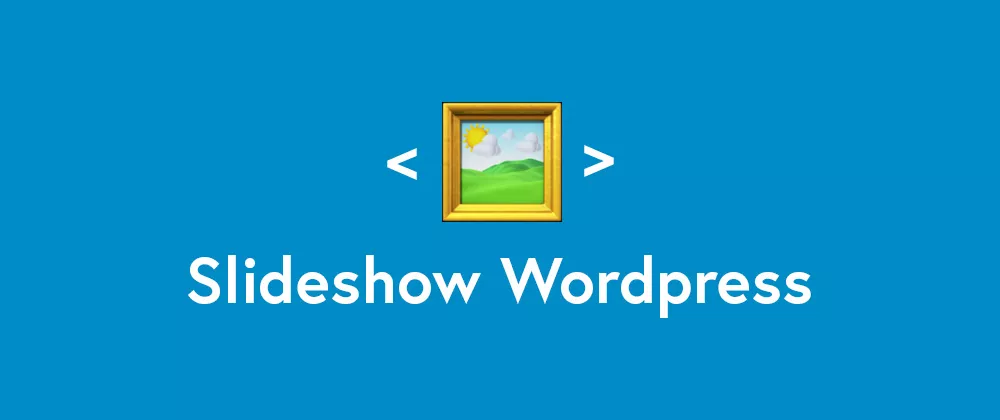
⏲ CreateHow to build a Wordpress slideshow in php with JavaScript and CSS
How to Build a WordPress Slideshow with PHP, JavaScript, and CSS
Hey there, slide enthusiasts! 👋 Ready to jazz up your WordPress site? Let's create a nifty slideshow without relying on plugins.
What We're Building
Picture this: a sleek slideshow showcasing your latest blog posts. It's like a catwalk for your content, but with less attitude and more automation.
The PHP and HTML Magic
First things first, let's grab those posts. We'll be working in your theme's front-page.php
. It's like the VIP entrance for your website's content.
<?php
$last_three_posts = new WP_Query('posts_per_page=3');
if ($last_three_posts->have_posts()) :
echo '<div id="slideshow">';
while ($last_three_posts->have_posts()) : $last_three_posts->the_post();
echo '<div class="mySlides fade">';
echo '<div id="numbertext"></div>';
echo '<img src="' . get_the_post_thumbnail_url() . '" alt="' . get_the_title() . '" style="width:100%">';
echo '<div id="text">' . get_the_title() . '</div>';
echo '</div>';
endwhile;
echo '<a id="previmg">❮</a>';
echo '<a id="nextimg">❯</a>';
echo '</div>';
wp_reset_postdata();
endif;
?>
This code is like a fishing net for your posts. It catches the last three and displays them in a fancy slideshow format.
Styling: Because Looks Matter
Let's make our slideshow look as good as a perfectly toasted marshmallow. Add this CSS to your style.css
:
.mySlides {
display: none;
}
.fade {
animation: fade 1.5s;
}
#slideshow {
position: relative;
max-width: 100%;
margin: auto;
}
#previmg, #nextimg {
cursor: pointer;
position: absolute;
top: 50%;
width: auto;
padding: 16px;
margin-top: -22px;
color: white;
font-weight: bold;
font-size: 18px;
transition: 0.6s ease;
border-radius: 0 3px 3px 0;
user-select: none;
background-color: rgba(0,0,0,0.8);
}
#nextimg {
right: 0;
border-radius: 3px 0 0 3px;
}
#text {
position: absolute;
bottom: 8px;
width: 100%;
text-align: center;
color: white;
background-color: rgba(0,0,0,0.5);
padding: 8px 0;
}
#numbertext {
position: absolute;
top: 0;
color: #f2f2f2;
padding: 8px 12px;
font-size: 12px;
}
@keyframes fade {
from {opacity: .4}
to {opacity: 1}
}
JavaScript
Now, let's add some interactivity.
document.addEventListener('DOMContentLoaded', function() {
let slideIndex = 0;
const slides = document.getElementsByClassName("mySlides");
const prevImg = document.getElementById('previmg');
const nextImg = document.getElementById('nextimg');
const numberText = document.getElementById('numbertext');
function showSlide(n) {
if (n >= slides.length) slideIndex = 0;
if (n < 0) slideIndex = slides.length - 1;
for (let i = 0; i < slides.length; i++) {
slides[i].style.display = "none";
}
slides[slideIndex].style.display = "block";
numberText.innerText = `${slideIndex + 1} / ${slides.length}`;
}
function nextSlide() {
showSlide(++slideIndex);
}
function prevSlide() {
showSlide(--slideIndex);
}
nextImg.addEventListener('click', nextSlide);
prevImg.addEventListener('click', prevSlide);
showSlide(slideIndex);
setInterval(nextSlide, 5000); // Auto-advance every 5 seconds
});
This script is the brains of the operation. It handles clicks, keeps track of which slide is showing, and even changes slides automatically. It's like having a tiny, invisible DJ for your images!
Wrapping Up
There you have it! A homemade WordPress slideshow that's cooler than a penguin's underwear. No plugins required, just pure coding goodness.
Remember, practice makes perfect. If your first attempt looks more like a slideshow of your aunt's blurry vacation photos, don't worry! Keep tweaking, and soon you'll have a slideshow smoother than a buttered dolphin.
Happy coding, and may your slides always be in focus!